Integrate Google Analytics into Your React Web-App (without npm packages)
Integrating an analytics solution is crucial for any business in order to measure success and where it originates from. Google Analytics is probably the most well known tool, so in this article you will learn how to use it in your React App without any npm packages.
In order to integrate Google Analytics into your React app, you first have to create the respective GA Property. Then copy the script that Google Analytics provides and add a script element into the body of your React app that contains the copied script at runtime.
After that, you can track different events and page views (even for single-page-applications) with ease. Let's dive into the details of how to do the above and more for your React web app.
But there is a React npm package for Google Analytics
There is an npm package for react which integrates Google Analytics and there is nothing wrong with using packages if they take off workload from you. In this case, though, the reduced workload is minimal and integrating the plain JavaScript Google Analytics version will yield the benefit of being well-documented.
If you go with the npm package, chances are that you run into issues (like me) and can't resolve them because there is only very limited resources about non-official npm packages.
If you go with the vanilla JavaScript version, however, you will be able to fix issues much faster as you can use all the resources on the internet about Google Analytics.
Everything should be done as simple as possible, but not simpler - Albert Einstein
At the end of the day, KISS (Keep it short and simple) is one of the clean code principles and adding yet another layer of complexity by adding an npm package shouldn't be the solution for this problem.
This is the code I use
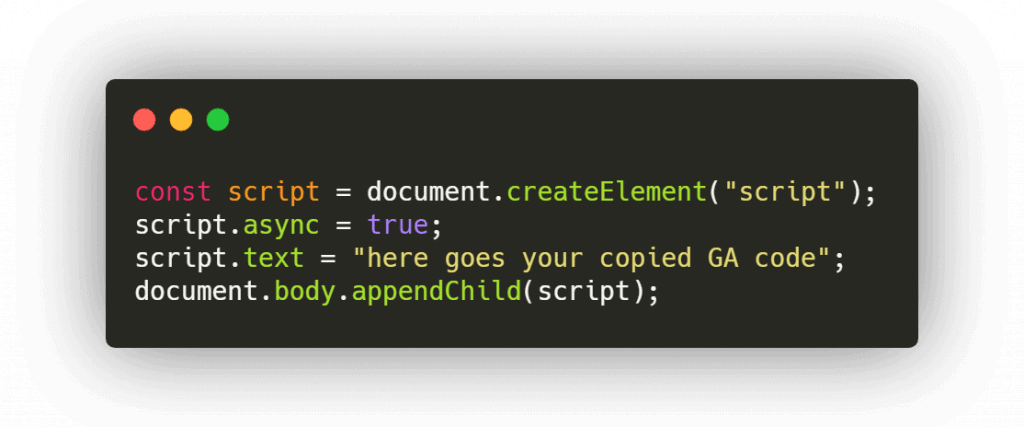
At its core I simply add a script element that contains the GA code to the body. The async
attribute is important if you don't want the page to stop parsing while executing the script.
I have this code snippet inside my top level component's componentDidMount
lifecycle method. It's that simple. This should do the trick for a very basic integration.
Only Track in Production
It's also worth mentioning that I only execute the above script when the code is running on production environment, so I don't record page views and events while I am developing.
I do this by having a different .env
on my production environment. If you don't know how to work with environment variables, I strongly recommend you to check out this great introduction and tutorial about environment variables. Be sure to search for react-specific solutions after reading the article, there are some caveats using env files with React.
Tracking Page Views Correctly
The above snippet does not work for capturing page views that do not execute a full page reload. As most React apps don't reload the page but only swap the displayed content, Google Analytics will only capture the first page view but not the consecutive page views produced by clicking inside your app.
To track page views correctly, we have to submit a page view event on each new page view. In order to do that, we need access to the gtag function that allows us to do so. I modified the copied Google Analytics code by adding a line after the gtag function definition:
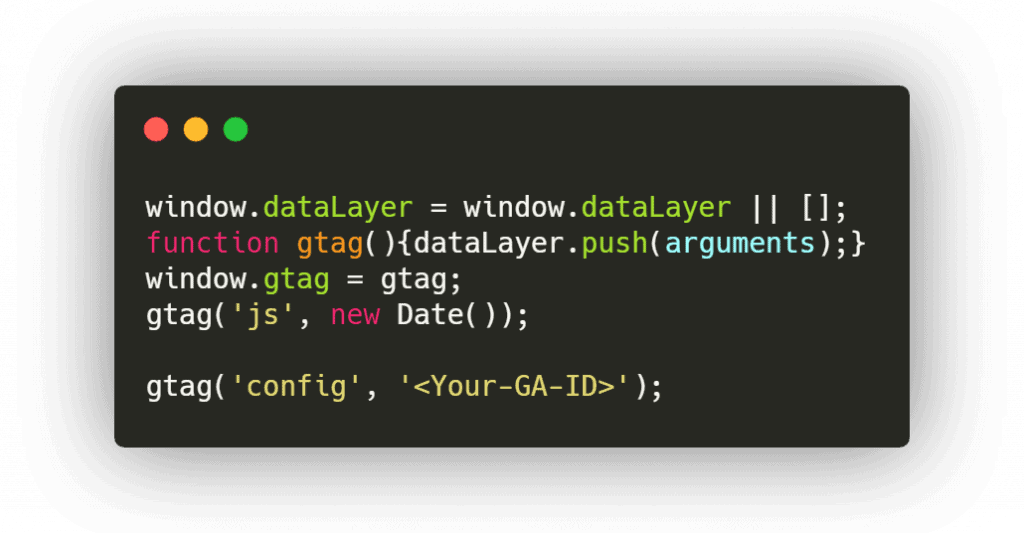
This way, the gtag function always available via window.gtag
. The following code sends a page view event "manually":
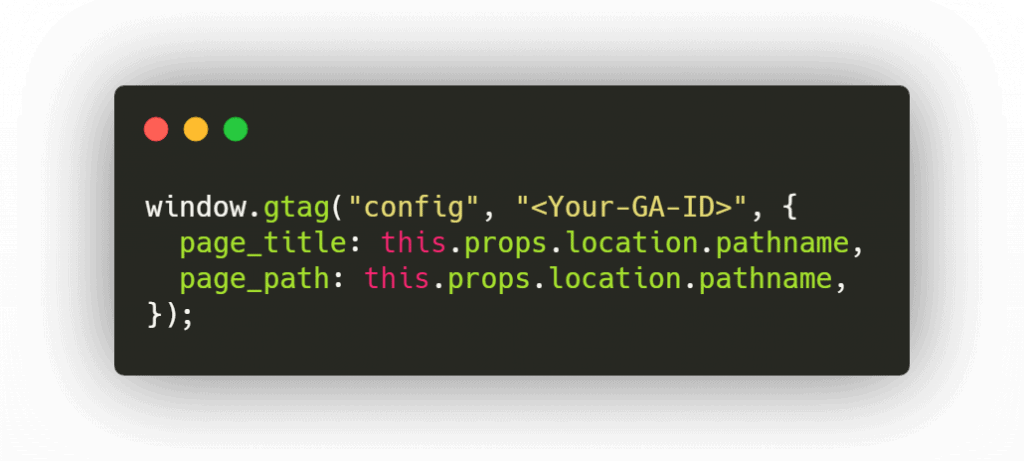
In my top level component, I execute this code when the location path changed. If it changed, I execute the above code. Use the componentDidUpdate
method with a little check to listen to location path changes:
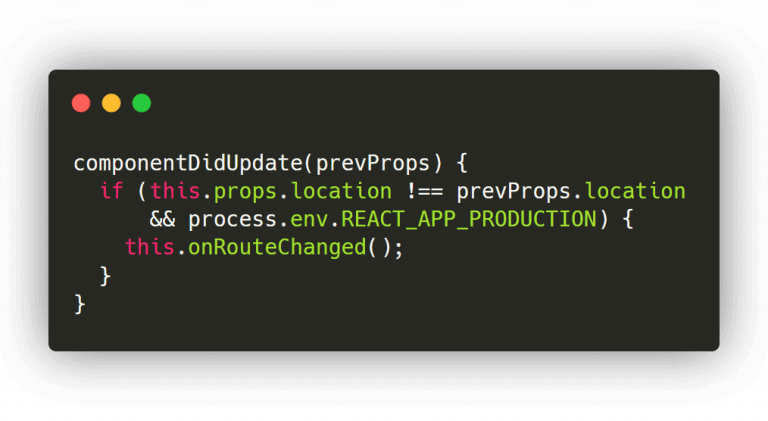
This will result in only sending a new page view if the location has changed and the code is running on the production environment.
Add Event Tracking
Let's get to the creative part. You'll probably already have a few ideas which events you want to capture but here are some ideas what to track to get you started:
- click on external URL events
- registration events
- login events
- sale events
- add to cart events
- subscription renewed event
Be sure to also track other business-specific events that are important for your website. You'll thank me later for having the data at hand when you need it (for example to see which channel brought the most sales and how different devices / countries are performing).
You simply have to add this line of code at any point where you want to trigger an event:
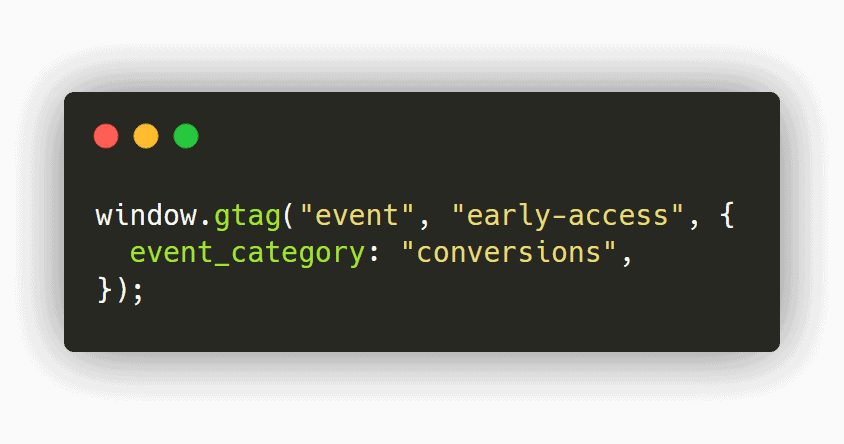
In this case, I send an event which has the action "early-access" and the event category "conversions". The good part about using the vanilla JS implementation is that you can simply go to the Google Analytics documentation and look how to for example specify a value or a label. Just be sure to use window.gtag
because gtag is not in the global scope.